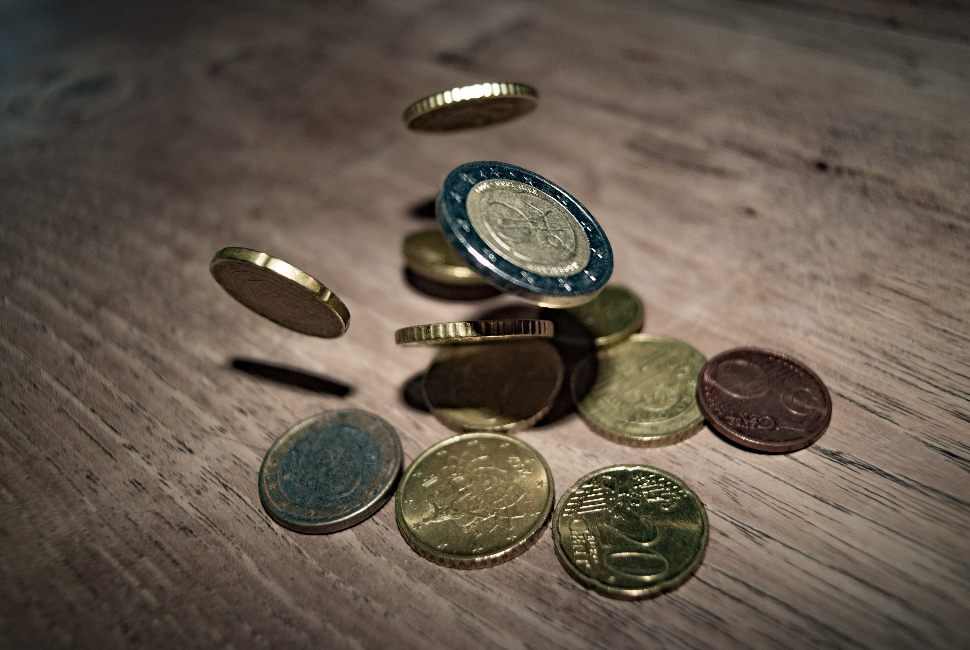
Expert Advisor Studio ~ Martingale EA
Tue Mar 03 2020
5 min read
Shanghai
# Expert Advisor Studio ~ Added a Martingale Expert Advisor to Fintechee.
Fintechee provides an open trading platform with SDK so-called expert advisor studio . Traders can code their own expert advisors at Fintechee.
# I think every advanced trader must have heard of Martingale algorithm.
Martingale algorithm makes you put more positions if the trend is opposite to the orientation that you trade. So sufficient funds would be a must to Martingale expert advisor.
I don't recommend traders use this algorithm. But it's interesting to watch how Martingale algorithms would be at Fintechee. And it's worth investigating. So I share the source code below with you. You can backtest it.
registerEA(
"sample_martingale",
"A test EA to show you how to get benefit from Martingale algorithm(v1.0)",
[{
name: "smaPeriod",
value: 5,
required: true,
type: "Integer",
range: [1, 100]
}, {
name: "smaPeriod2",
value: 7,
required: true,
type: "Integer",
range: [1, 100]
}, {
name: "symbol",
value: "EUR/USD",
required: true,
type: "String",
range: null
}, {
name: "timeFrame",
value: TIME_FRAME.H1,
required: true,
type: "String",
range: null
}, {
name: "volume",
value: 0.01,
required: true,
type: "Number",
range: [0, 100000]
}, {
name: "diff",
value: 0.001,
required: true,
type: "Number",
range: [0, 100000]
}, {
name: "step",
value: 0.001,
required: true,
type: "Number",
range: [0, 100000]
}],
function (context) { // Init()
var account = getAccount(context, 0)
var brokerName = getBrokerNameOfAccount(account)
var accountId = getAccountIdOfAccount(account)
var symbolName = getEAParameter(context, "symbol")
var timeFrame = getEAParameter(context, "timeFrame")
var smaPeriod = getEAParameter(context, "smaPeriod")
var smaPeriod2 = getEAParameter(context, "smaPeriod2")
getQuotes(context, brokerName, accountId, symbolName)
window.chartHandle = getChartHandle(context, brokerName, accountId, symbolName, timeFrame)
window.smaHandle = getIndicatorHandle(context, brokerName, accountId, symbolName, timeFrame, "sma", [{
name: "period",
value: smaPeriod
}])
window.smaHandle2 = getIndicatorHandle(context, brokerName, accountId, symbolName, timeFrame, "sma", [{
name: "period",
value: smaPeriod2
}])
},
function (context) { // Deinit()
delete window.currTime
delete window.smaHandle
delete window.smaHandle2
},
function (context) { // OnTick()
var arrTime = getData(context, window.chartHandle, DATA_NAME.TIME)
if (typeof window.currTime == "undefined") {
window.currTime = arrTime[arrTime.length - 1]
} else if (window.currTime != arrTime[arrTime.length - 1]) {
window.currTime = arrTime[arrTime.length - 1]
} else {
return
}
var account = getAccount(context, 0)
var brokerName = getBrokerNameOfAccount(account)
var accountId = getAccountIdOfAccount(account)
var symbolName = getEAParameter(context, "symbol")
var volume = getEAParameter(context, "volume")
var diff = getEAParameter(context, "diff")
var step = getEAParameter(context, "step")
var arrSma = getData(context, window.smaHandle, "sma")
var arrSma2 = getData(context, window.smaHandle2, "sma")
var ask = getAsk(context, brokerName, accountId, symbolName)
var bid = getBid(context, brokerName, accountId, symbolName)
var checkOpenTrd = function (price, op) {
var openTradeLength = getOpenTradesListLength(context)
var profit = 0
var count = 0
for (var i = openTradeLength - 1; i >= 0; i--) {
var openTrade = getOpenTrade(context, i)
if (getOrderType(openTrade) == op) {
profit += getUnrealizedPL(openTrade)
}
}
if (profit > diff) {
for (var i = openTradeLength - 1; i >= 0; i--) {
var openTrade = getOpenTrade(context, i)
if (getOrderType(openTrade) == op) {
var tradeId = getTradeId(openTrade)
closeTrade(brokerName, accountId, tradeId)
count++
}
}
return count
}
return 0
}
var checkAndSendOrder = function (price) {
var openTradeLength = getOpenTradesListLength(context)
var lowestPrice = 0
var highestPrice = 0
var longCount = 0
var shortCount = 0
for (var i = openTradeLength - 1; i >= 0; i--) {
var openTrade = getOpenTrade(context, i)
var openPrice = getOpenPrice(openTrade)
var type = getOrderType(openTrade)
if (type == ORDER_TYPE.OP_BUY) {
if (lowestPrice == 0) {
lowestPrice = openPrice
} else if (openPrice < lowestPrice) {
lowestPrice = openPrice
}
longCount++
} else {
if (highestPrice == 0) {
highestPrice = openPrice
} else if (openPrice > highestPrice) {
highestPrice = openPrice
}
shortCount++
}
}
if (lowestPrice != 0 && lowestPrice - price > step) {
sendOrder(brokerName, accountId, symbolName, ORDER_TYPE.OP_BUY, 0, 0, volume, 0, 0, "")
return longCount + 1
}
if (highestPrice != 0 && price - highestPrice > step) {
sendOrder(brokerName, accountId, symbolName, ORDER_TYPE.OP_SELL, 0, 0, volume, 0, 0, "")
return shortCount + 1
}
if (lowestPrice != 0) {
return longCount
}
if (highestPrice != 0) {
return shortCount
}
if (op == ORDER_TYPE.OP_BUY) {
sendOrder(brokerName, accountId, symbolName, ORDER_TYPE.OP_BUY, 0, 0, volume, 0, 0, "")
} else {
sendOrder(brokerName, accountId, symbolName, ORDER_TYPE.OP_SELL, 0, 0, volume, 0, 0, "")
}
return 1
}
var op = null
if (arrSma[arrSma.length - 1] > arrSma2[arrSma2.length - 1]) {
op = ORDER_TYPE.OP_BUY
} else {
op = ORDER_TYPE.OP_SELL
}
if (op == ORDER_TYPE.OP_BUY) {
checkOpenTrd(bid, ORDER_TYPE.OP_BUY)
checkAndSendOrder(ask, ORDER_TYPE.OP_BUY)
} else if (op == ORDER_TYPE.OP_SELL) {
checkOpenTrd(ask, ORDER_TYPE.OP_SELL)
checkAndSendOrder(bid, ORDER_TYPE.OP_SELL)
}
})
We started trading since Feb 17th, 2020. Please feel free to track our trading records.
- Fintechee WEB Trader
- Account ID: 875730
- Investor Password: 1